Stateless vs. Stateful Widgets in Flutter: The Foundation of Your User Interface
In Flutter, everything you see on the screen is a Widget.
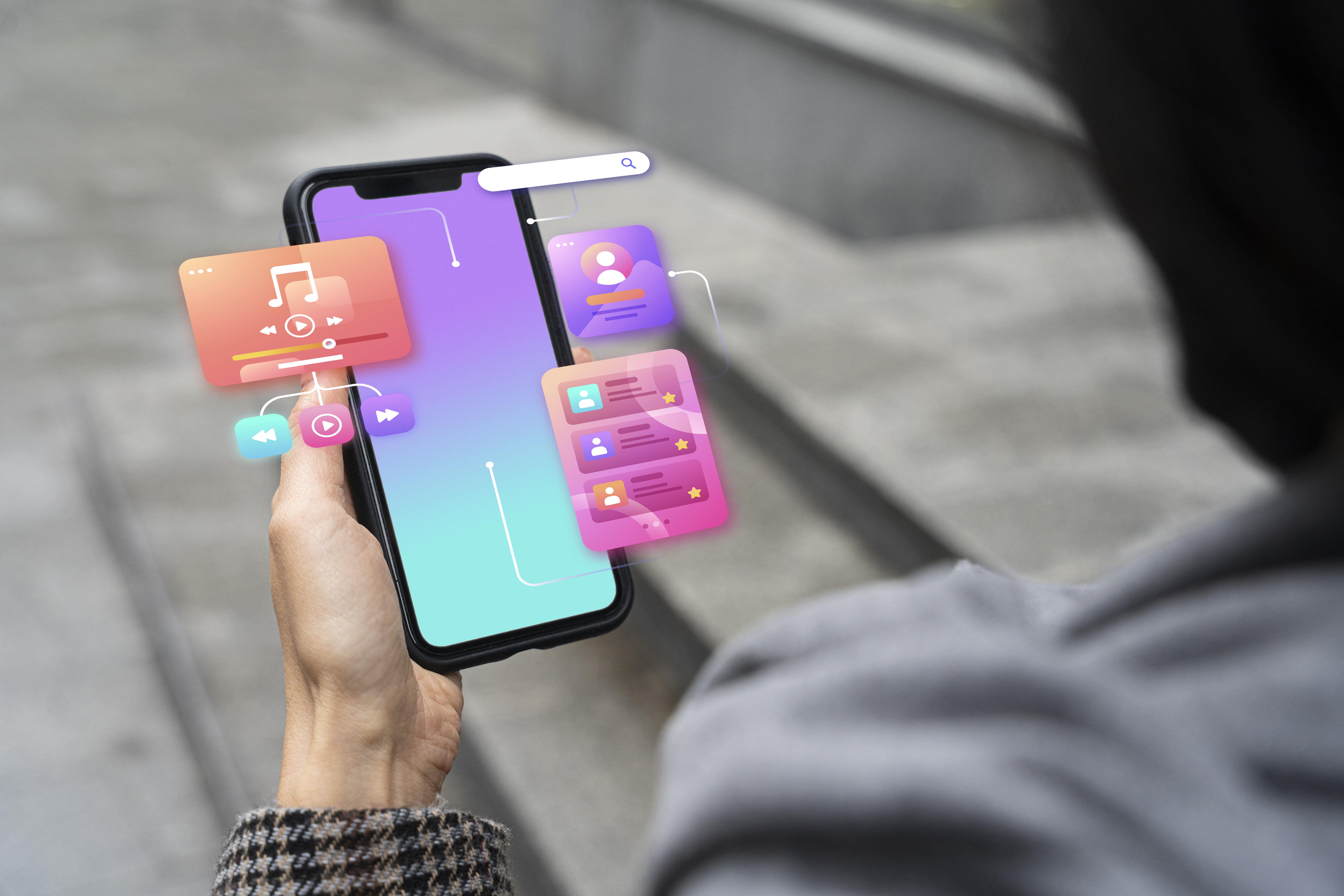
Introduction
Widgets are the fundamental building blocks, defining the structure and appearance of your user interface (UI). Understanding the difference between Stateless and Stateful Widgets is essential for building effective and dynamic Flutter applications.
Stateless Widgets
- Immutable Stateless Widgets are immutable, meaning their properties cannot be changed once they are created. Think of them like a photograph – once taken, it cannot be altered.
- Simple and Efficient: They are ideal for static UI elements, such as text, icons, or images that do not change during the app's runtime.
Example
class SimpleText extends StatelessWidget {
final String text;
SimpleText({required this.text});
@override
Widget build(BuildContext context) {
return Text(text);
}
}
Stateful Widgets
- Mutable: Stateful Widgets hold an internal state that can change over time, for instance, in response to user interaction or external events.
- Dynamic: They are necessary for interactive UI elements, such as buttons, text fields, or sliders, which can alter their appearance or behavior.
Example
class InteractiveButton extends StatefulWidget {
@override
_InteractiveButtonState createState() =>
_InteractiveButtonState();
}
class _InteractiveButtonState extends State {
bool _isPressed = false;
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: () {
setState(() {
_isPressed = !_isPressed;
});
},
child: Container(
padding: EdgeInsets.all(16.0),
decoration: BoxDecoration(
color: _isPressed ? Colors.blue : Colors.grey,
borderRadius: BorderRadius.circular(8.0),
),
child: Text(
'Press Me',
style: TextStyle(color: Colors.white),
),
),
);
}
}
How They Work Together
- Widget Tree: Flutter constructs a hierarchy of Widgets, called a Widget tree, to represent the UI.
- Rebuilding: When the state of a Stateful Widget changes, Flutter automatically rebuilds the relevant portion of the Widget tree to reflect the change. Stateless Widgets are only rebuilt if their properties are modified.
- Efficiency: Flutter employs a smart reconciliation mechanism to minimize the number of rebuilds and optimize performance.
When to Use Which
- Stateless: Use Stateless Widgets for static UI elements that do not change over time.
- Stateful: Use Stateful Widgets for interactive or dynamic UI elements that require updates.
Best Practices for UI Construction
- Composition over Inheritance: Favor composing complex UIs by combining smaller, simpler Widgets instead of creating large, monolithic ones. This promotes reusability and maintainability..
- Stateless by Default: Start with Stateless Widgets whenever possible and only introduce Stateful Widgets when necessary. This improves performance and reduces complexity.
- Optimize Rebuilds: Be mindful of how often your Widgets rebuild. Use const constructors for Widgets that don't change and consider using const for specific parts of your Widget tree to prevent unnecessary rebuilds.
- Use setState Carefully: setState({}) will cause the Widget to rebuild. For increased performance, pages should be Stateless and include Stateful Widgets. Keep setState as simple as possible to avoid unnecessary reloads.
Conclusion
Understanding the distinction between Stateless and Stateful Widgets is fundamental to Flutter development. Choosing the right Widget type ensures an efficient, dynamic, and performant user interface. By following best practices for UI construction, you can create maintainable and scalable Flutter applications.