Android Jetpack Compose: New Type Safety Navigation
Navigation is a cornerstone of every Android application.
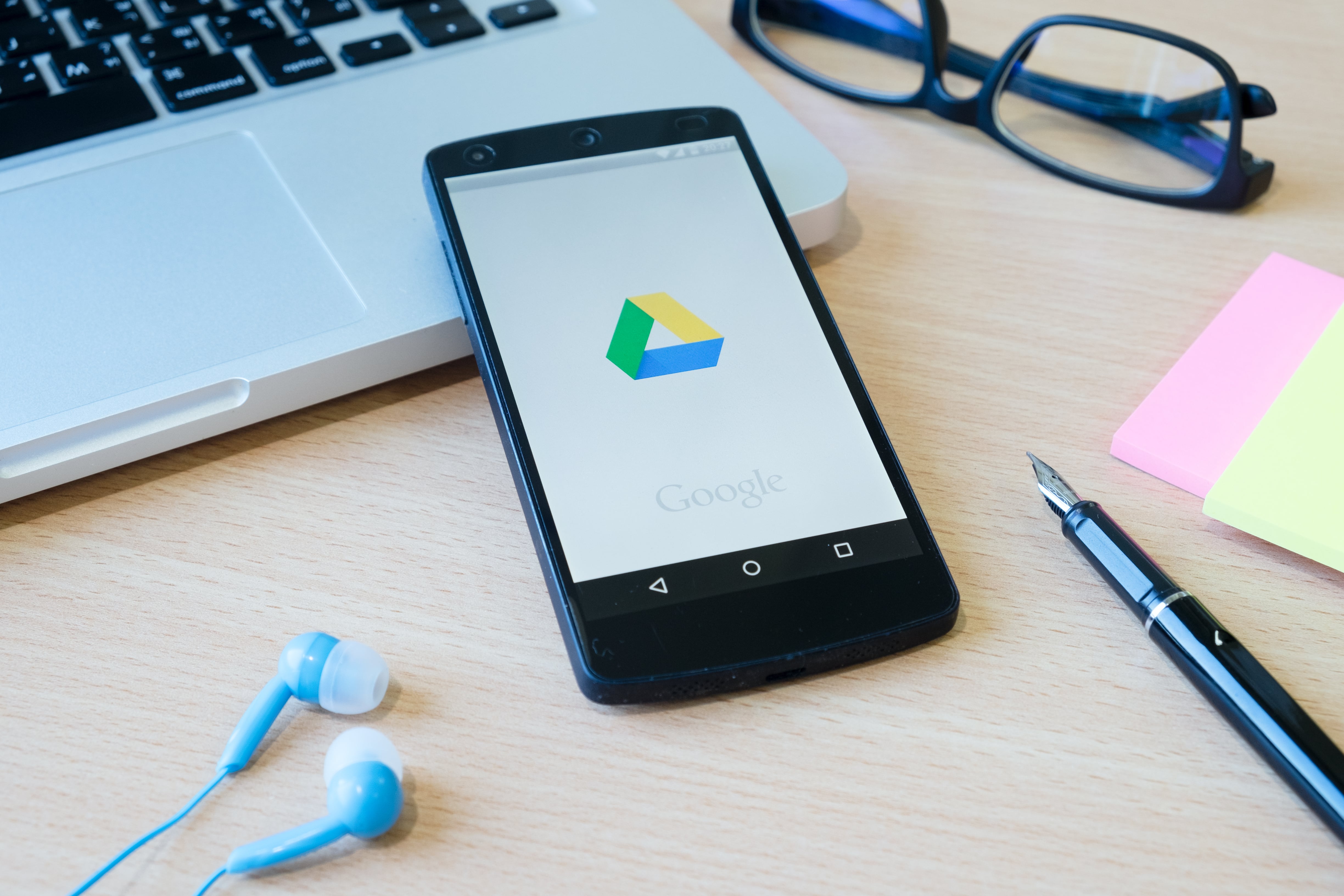
Introduction
With the introduction of type-safe navigation in Jetpack Compose, developers can enjoy cleaner code, fewer bugs, and improved debugging capabilities. This article explores how to leverage @Serializable data classes to pass arguments between screens, replacing less robust navigation methods of the past.
Why Type-Safe Navigation?
Traditional navigation methods rely on manually passing arguments as strings, which can lead to runtime errors due to incorrect types or missing parameters. Type-safe navigation solves these issues by ensuring compile-time verification of navigation arguments. This approach:
- Reduces runtime crashes caused by incorrect argument passing.
- Improves code readability and maintainability.
- Enhances debugging capabilities by ensuring strict type checks.
Implementing Type-Safe Navigation in Jetpack Compose
Let's walk through a practical example where we implement a custom ProductPage using type-safe navigation with @Serializable data classes.
1. Define the ProductPage Composable:
@Composable
fun ProductPage(
modifier: Modifier = Modifier,
routeArgs: ProductRoute,
viewModel:
ProductViewModel = hiltViewModel()
) {
...
}
The ProductPage composable receives ProductRoute as a parameter, ensuring that all required arguments are type-safe and easily accessible
2. Define the @Serializable Data Class
@Serializable
data class ProductRoute(
val productId: String,
val name: String?,
val category: String?,
val isWishList: Boolean,
)
This data class holds the arguments required for navigation. Marking it with @Serializable ensures seamless argument passing.
3. Define a custom navigation extension function
fun NavController.navigateToProduct(
productId: String,
name: String? = null,
category: String? = null,
isWishList: Boolean = true,
) = navigate(
route = ProductRoute(
productId = productId,
name = name,
category = category,
isWishList = isWishList
)
)
This code defines a handy shortcut (an extension function) called navigateToProduct for navigating in your app. Instead of writing a long navigation command every time, you can now just call navController.navigateToProduct() and give it the product details. It automatically builds the correct navigation path for you, including the product ID, name, category, and wishlist status. This makes your code cleaner and less error-prone.
4. Set up how to navigate to a screen
fun NavGraphBuilder.productNavigation() {
composable {
val arg = it.toRoute()
ProductPage(routeArgs = arg)
}
}
This code sets up how to navigate to ProductPage in the app. It says "when navigating to the ProductRoute, grab the product info (arguments) and show the ProductPage with that info." The <ProductRoute> and toRoute() part ensures this is done safely, with the correct data types.
5. Add the custom navigation to your NavHost
NavHost(
modifier = modifier,
navController = appState.navController,
) {
screenA()
productNavigation()
screenB() {
screenB1()
screenB2()
}
}
The productNavigation() function is now integrated into the NavHost, making ProductPage accessible within the app's navigation structure.
6. Navigate to ProductPage
navController.navigateToProductListing(
categoryId = "AN0R31",
name = "Milk"
)
With this setup, you can safely navigate to ProductPage from anywhere in your app with a concise and type-safe approach.
Debugging and Testing Type-Safe Navigation?
When implementing type-safe navigation, testing is crucial to ensure everything works correctly. Some best practices include:
- Using unit tests to validate the serialization and deserialization of navigation arguments.
- Verifying navigation behavior with UI tests in Jetpack Compose.
- Leveraging logcat debugging to inspect passed arguments in case of unexpected behavior.
Conclusion
Type-safe navigation in Jetpack Compose significantly improves code maintainability, readability, and reliability.
By leveraging @Serializable data classes and custom navigation functions, developers can create robust navigation flows with minimal effort.
Additionally, integrating debugging and testing strategies ensures smooth navigation experiences in production applications.
Start implementing this approach in your projects today for a more structured and error-free navigation experience!