Mastering Navigation in Flutter: A Deep Dive into GoRouter with Enum-Based Routing
Navigation is a critical aspect of building mobile applications
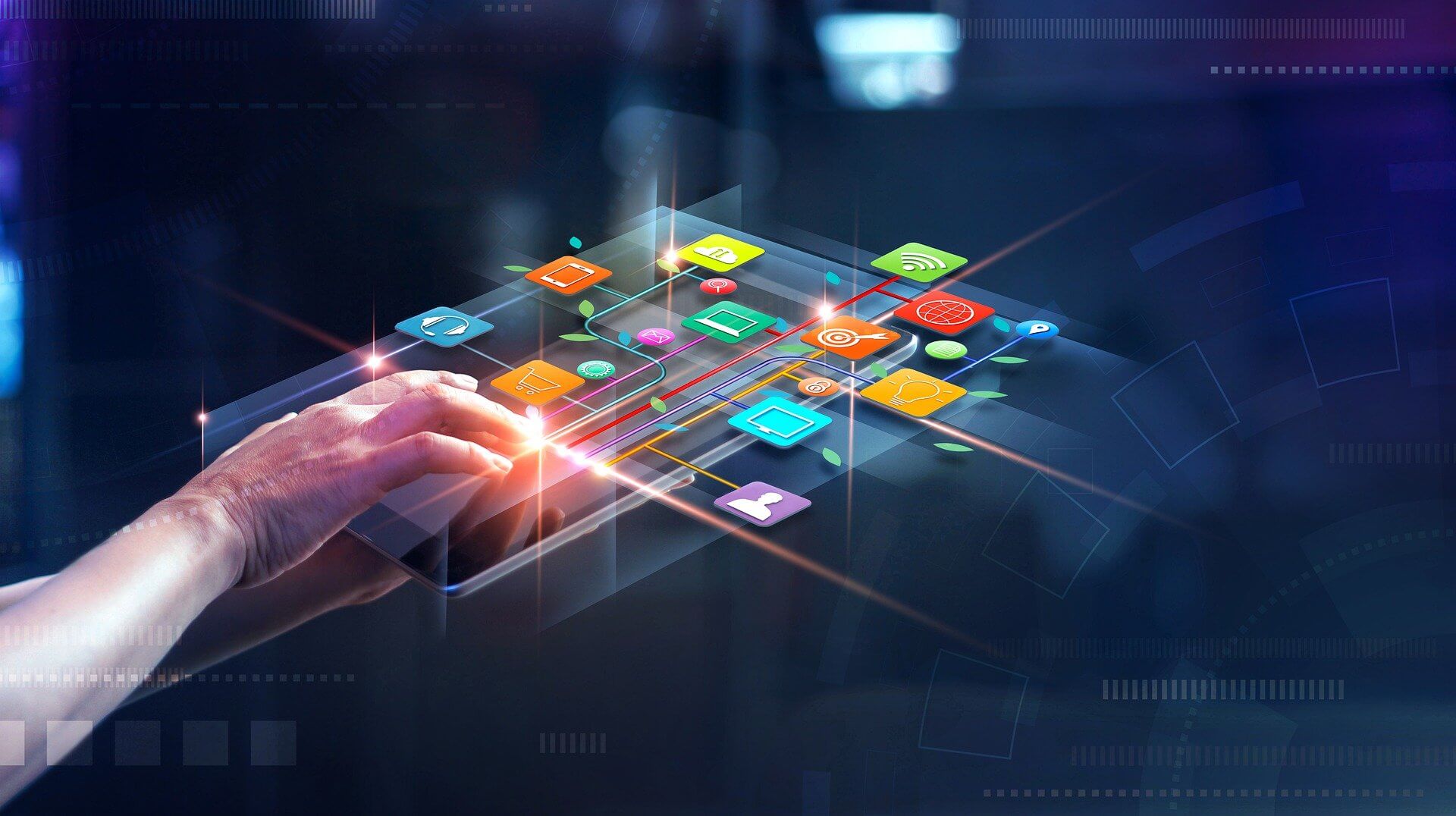
Introduction
Navigation is a critical aspect of building mobile applications, and as your Flutter app grows in
complexity, managing routes efficiently becomes more important. GoRouter
is an excellent
routing solution in Flutter, offering a clean and declarative way to handle navigation. By
combining GoRouter
with an enum-based routing strategy, you can simplify your navigation
code, make it more maintainable, and reduce errors. This article will explore how to use
GoRouter
with an enum class to manage routes, providing a practical and scalable approach to
building robust navigation in Flutter apps.
The Need for Robust Routing
As Flutter apps become more feature-rich, managing navigation can become a challenging task.
Traditional approaches to routing often lead to scattered or tangled code, making it difficult to
scale the application or maintain a consistent navigation experience. GoRouter
addresses these
challenges by offering a declarative approach to defining and managing routes, making it easier
to understand and maintain. When combined with an enum-based approach, GoRouter
makes
routing even more structured and centralized, allowing for better maintainability and scalability.
Setting Up GoRouter with Enum-Based Routes
A great way to manage your routes is by using an enum class. This approach centralizes all the
route definitions and ensures that route paths are consistent throughout your app. Below, we'll
walk through the steps to set up GoRouter
with an enum class in your Flutter project.
The first step is to define an enum class called RoutePath. Each entry in the enum will represent a route in your app, and you can easily associate each route with a path and, optionally, a name. Here’s an example of how to define this enum:
enum RoutePath {
// Routes with absolute paths (start with '/')
root(path: '/'),
home(path: '/home'),
search(path: '/search'),
profile'(path: '/profile'),
// Routes with relative paths (no leading '/')
settings(path: 'settings'),
edit(path; 'edit'),
// Add new routes above this line
;
const RoutePath({required this.path});
final String path;
// Optionally, we can provide a computed 'name' property
String get name => this.toString().split('.').last;
}
- RoutePath Enum: This enum holds all route definitions in a single place, making it easy to update and manage.
- path: Each enum constant holds a path value, which is the route's URL pattern.
- name: The name property uses the enum’s name to give a unique identifier to each route, avoiding hardcoded strings in the app and improving code readability.
Step 2: Set Up GoRouter with Enum Routes
Now that we have our enum defined, let's set up GoRouter to use it for defining routes in the app. This will centralize the routing logic and ensure consistency throughout the app.
final _router = GoRouter(
routes: [
// Root route
GoRoute(
path: RoutePath.root.path,
// Using enum for route name
name: RoutePath.root.name,
builder: (context, state) => const RootScreen(),
),
// Home route
GoRoute(
path: RoutePath.home.path,
// Using enum for route name
name: RoutePath.home.name,
builder: (context, state) => const HomeScreen(),
),
// Search route
GoRoute(
path: RoutePath.search.path,
// Using enum for route name
name: RoutePath.search.name,
builder: (context, state) => const SearchScreen(),
),
],
);
- path and name: We use
RoutePath.enumName.path
andRoutePath.enumName.name
to ensure route paths and names are consistently used across the app. - Builder Functions: The
builder
functions define what widget to display when a specific route is triggered. For the/announcement/:data
route, we access the dynamic data parameter usingstate.extra
.
Step 3: Configuring GoRouter in MaterialApps
To hook up your GoRouter configuration into your app, use the routerConfig
property in the MaterialApp.router
widget:
MaterialApp.router(
routerConfig: _router,
// Other properties like theme, locale, etc.
);
This tells Flutter to use the router configuration you defined in the previous step.
Navigating with GoRouter
GoRouter provides several methods to navigate between screens, allowing you to handle navigation in a structured and declarative way.
Navigating by Path
You can navigate to a route by specifying the path
directly. Here's an example:
// Navigate to Home screen
GoRouter.of(context).go(RoutePath.home.path);
This method completely replaces the current route with the new one.
Navigating Using Named Routes
You can also use named routes for navigation, which helps make your code more maintainable:
GoRouter.of(context).goNamed(
RoutePath.announcement.name,
params: {'data': 'announcementData'}
);
Using RoutePath.announcement.name
ensures that the name of the route is always consistent, which reduces the risk of errors when dealing with navigation.
Navigating with Parameters
For routes that include dynamic parameters (e.g., /announcement/:data
), you can pass data in the URL, and GoRouter provides an easy way to access it:
GoRoute(
path: RoutePath.announcement.path,
name: RoutePath.announcement.name,
builder: (context, state) {
final data = state.extra! as HomePageAnnouncementModel;
return AnnouncementPage(announcement: data);
},
);
The state.extra
property is used to pass and retrieve data when navigating to routes with dynamic segments.
Handling Nested Routes
GoRouter supports nested routing, which allows you to structure your app with hierarchical routes. This is especially useful for apps with tab navigation or nested screens.
GoRoute(
path: RoutePath.profile.path,
builder: (context, state) => const ProfileScreen(),
routes: [
GoRoute(
path: RoutePath.settings.path,
builder: (context, state)
=> const SettingsScreen(),
),
GoRoute(
path: RoutePath.edit.path,
builder: (context, state)
=> const EditProfileScreen(),
),
],
);
In this example, the ProfileScreen
is the parent route, and SettingsScreen
and EditProfileScreen
are nested within it. Nested routes allow for a more organized and scalable route structure.
Redirecting Users Based on Conditions
GoRouter also supports conditional redirects. You can redirect users based on certain conditions, such as authentication or other global states:
GoRouter(
// Trigger redirection on changes
refreshListenable: someListenable,
redirect: (BuildContext context, GoRouterState state) {
if (!isAuthenticated) {
// Redirect to root if not authenticated
return RoutePath.root.path;
}
// No redirect
return null;
},
routes: [...],
);
In this case, users are redirected to the root route if they are not authenticated.
Custom Transitions
GoRouter allows you to define custom transitions for your routes, which can be useful for creating unique animations between screens:
GoRoute(
path: RoutePath.home.path,
name: RoutePath.home.name,
pageBuilder: (context, state)
=> CustomTransitionPage(
key: state.pageKey,
child: const HomeScreen(),
),
);
class CustomTransitionPage
extends CustomTransitionBuilder {
@override
Widget buildTransition(
BuildContext context,
Animation animation,
Animation secondaryAnimation,
Widget child,
) {
return FadeTransition(
opacity: animation,
child: child
);
}
}
This example uses a FadeTransition
to animate the transition between screens.
Conclusion
GoRouter, combined with an enum-based routing system, offers a powerful, flexible, and scalable solution for managing navigation in Flutter apps. By centralizing route definitions in an enum, you reduce the risk of errors and improve the maintainability of your code. With GoRouter's support for features like nested routes, named routes, and custom transitions, you can create a robust and user-friendly navigation experience for your app.
By using GoRouter with enums, you’ll achieve cleaner, more structured routing logic that scales as your app grows. Don't forget to check the official GoRouter documentation for updates and more advanced features that can further enhance your navigation experience.