Flutter State Management Provider vs. Riverpod vs. BLoC
In this article, we will explore three popular state management solutions in Flutter: Provider, Riverpod, and BLoC
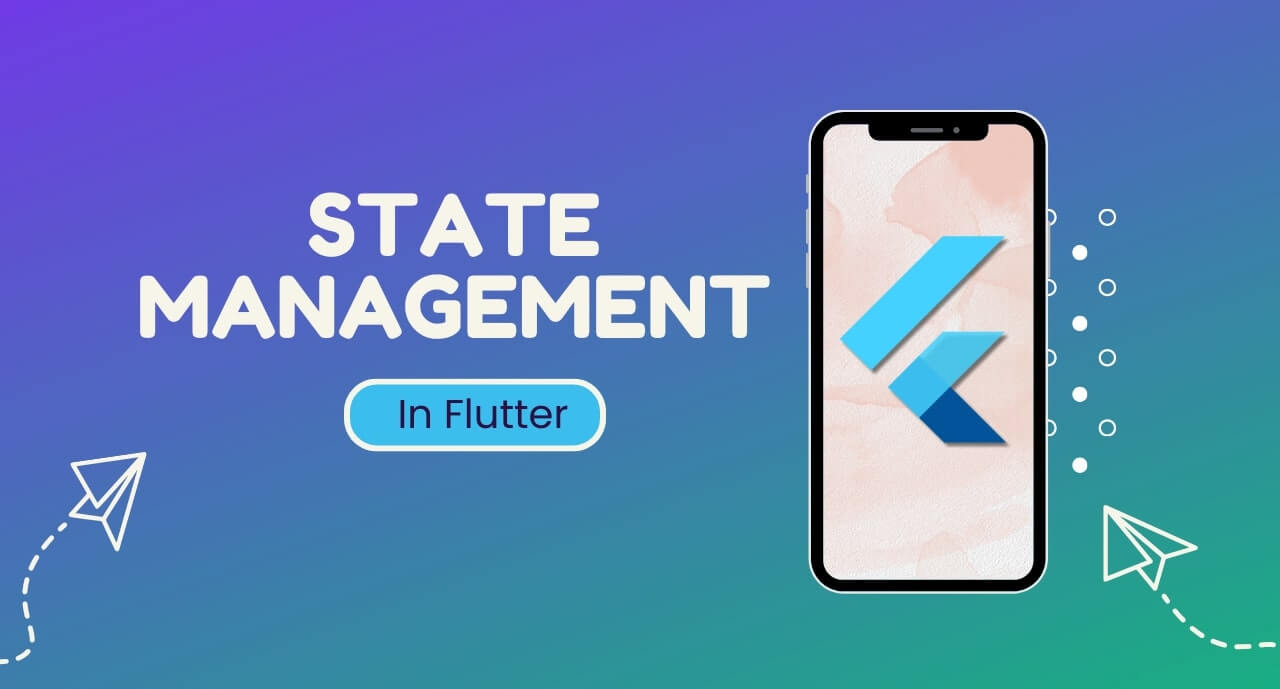
Introduction
In this article, we will explore three popular state management solutions in Flutter: Provider, Riverpod, and BLoC. We will discuss their strengths, weaknesses, and use cases to help you choose the best approach for your next Flutter project.
What is Flutter? - Building Cross-Platform Apps with a Single Codebase
Flutter, an open-source mobile SDK (Software Development Kit) crafted by Google, has rapidly become a favorite for developers aiming to create high-performance and visually stunning applications. Its core strength lies in its ability to build truly cross-platform apps for mobile (iOS and Android), web (Flutter web), and desktop (Flutter desktop) using a single codebase. This "write once, deploy anywhere" philosophy significantly reduces development time and resources, making Flutter an attractive option for startups, enterprises, and individual developers alike.
In this article, we will explore three popular state management solutions in Flutter: Provider, Riverpod, and BLoC. We will discuss their strengths, weaknesses, and use cases to help you choose the best approach for your next Flutter project.
Provider
Flutter Provider is a powerful state management library for Flutter. It makes it easy to manage the state of your application and share it between widgets.
What are Providers?
Providers are objects that hold and manage the state of your application. They are similar to global variables, but they are more flexible and efficient. Providers can be used to share data between widgets, to listen for changes to the state of the application, and to rebuild widgets when the state changes.
There are two main types of providers in Flutter:
- ChangeNotifierProviders: These providers notify the Flutter framework when their state changes. This allows the framework to rebuild widgets that depend on the state.
- ValueNotifierProviders: These providers are similar to ChangeNotifierProviders, but they only notify the Flutter framework when their value changes.
How to use Providers
To use a Provider, you first need to create a new provider class. The class should extend the ChangeNotifier
or ValueNotifier
class. Then, you can create a new instance of the provider class and pass it to the Provider
widget.
class MyProvider extends ChangeNotifier {
int _counter = 0;
int get counter => _counter;
void incrementCounter() {
_counter++;
notifyListeners();
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Provider(
create: (context) => MyProvider(),
child: MaterialApp(
home: MyHomePage(),
),
);
}
}
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Consumer(
builder: (context, provider, _) {
return Text('Counter: ${provider.counter}');
},
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
Provider.of(context).incrementCounter();
},
child: Icon(Icons.add),
),
);
}
}
In the example above, we create a new MyProvider
class that extends the ChangeNotifier
class. We then create a new instance of the MyProvider
class and pass it to the Provider widget. The Provider
widget is used to make the MyProvider
instance available to all widgets in the app.
The MyHomePage
widget uses the Consumer
widget to access the MyProvider
instance. The Consumer
widget rebuilds the widget whenever the state of the MyProvider
instance changes.
How to use the Selector widget
The Selector
widget is a more efficient way to rebuild widgets that depend on the state of a provider. The Selector
widget only rebuilds the widget when the specific part of the state that the widget depends on changes.
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Selector(
selector: (provider, _) => provider.counter,
builder: (context, counter, _) {
return Text('Counter: $counter');
},
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
Provider.of(context).incrementCounter();
},
child: Icon(Icons.add),
),
);
}
}
In the example above, we use the Selector
widget to rebuild the Text
widget only when the counter
property of the MyProvider
instance changes.
Flutter Provider is a powerful and flexible state management library for Flutter. It makes it easy to manage the state of your application and share it between widgets. By using the Provider
and Selector
widgets, you can write more efficient and maintainable Flutter code.
Riverpod: Simplifying State Management in Flutter
Flutter, Google's UI toolkit, empowers developers to build beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. A crucial aspect of any robust Flutter application is effective state management. Riverpod, a powerful and type-safe state management solution, builds upon the concepts of Provider while addressing some of its limitations. This article explores the core concepts of Riverpod and how it simplifies state management in Flutter.
Why Riverpod? Addressing the Challenges of Provider
While Provider offers a straightforward way to manage state, it can encounter challenges in larger applications, particularly with testability and compile-time safety. Riverpod tackles these issues head-on:
- Compile-Time Safety: Riverpod leverages compile-time code generation, ensuring that state management errors are caught during development rather than at runtime. This significantly reduces the risk of unexpected bugs and improves application stability.
- Improved Testability: Riverpod's architecture facilitates writing unit tests for your state management logic. Providers can be easily mocked and overridden in tests, making it simpler to isolate and verify the behavior of your application's state.
- Global Access Without Global State: Riverpod provides a way to access state anywhere in your widget tree without relying on inherently problematic global state. This promotes better code organization and maintainability.
Core Concepts: Providers, Ref, and Scoping
Riverpod introduces several key concepts that underpin its approach to state management:
- Providers: At the heart of Riverpod are providers. These are objects that hold and manage state. Unlike Provider, Riverpod's providers are globally accessible but do not rely on global state. They are defined using code generation, ensuring type safety. Examples include
StateProvider
(for simple state),ChangeNotifierProvider
(for state that needs to notify listeners), andFutureProvider
(for asynchronous data). ref
(ProviderContainer): Theref
object is your gateway to interacting with providers. It provides access to the current state held by a provider.ref
is available within widgets using theConsumer
orConsumerWidget
and can also be passed around as needed.- ProviderScope: The
ProviderScope
is a widget that makes providers available to the widget tree. It should be placed at the root of your application. This scope manages the lifecycle of providers.
A Simple Example: Counter App with Riverpod
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
final counterProvider = StateProvider((ref) => 0);
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// Wrap your app with ProviderScope
return ProviderScope(
child: MaterialApp(
home: MyHomePage(),
),
);
}
}
// Use ConsumerWidget for easy access to providers
class MyHomePage extends ConsumerWidget {
@override
Widget build(BuildContext context, WidgetRef ref) {
// Access the counter state using ref.watch
final counter = ref.watch(counterProvider);
return Scaffold(
appBar: AppBar(title: Text('Riverpod Counter')),
body: Center(child: Text('Counter: $counter')),
floatingActionButton: FloatingActionButton(
onPressed: () {
// Update the counter state using ref.read
ref.read(counterProvider.notifier).state++;
},
child: Icon(Icons.add),
),
);
}
}
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
final counterProvider = StateProvider((ref) => 0);
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// Wrap your app with ProviderScope
return ProviderScope(
child: MaterialApp(
home: MyHomePage(),
),
);
}
}
// Use ConsumerWidget for easy access to providers
class MyHomePage extends ConsumerWidget {
@override
Widget build(BuildContext context, WidgetRef ref) {
// Access the counter state using ref.watch
final counter = ref.watch(counterProvider);
return Scaffold(
appBar: AppBar(title: Text('Riverpod Counter')),
body: Center(child: Text('Counter: $counter')),
floatingActionButton: FloatingActionButton(
onPressed: () {
// Update the counter state using ref.read
ref.read(counterProvider.notifier).state++;
},
child: Icon(Icons.add),
),
);
}
}
Explanation:
- We define a
StateProvider
called counterProvider
to hold the counter's integer value.
- The
MyApp
is wrapped with ProviderScope
, making the providers available.
MyHomePage
uses ConsumerWidget to easily access providers.
ref.watch(counterProvider)
listens to changes in the counterProvider
and rebuilds the widget when the counter value updates.
ref.read(counterProvider.notifier).state++
reads the provider and increments the state, triggering a rebuild due to ref.watch.
StateProvider
called counterProvider
to hold the counter's integer value.MyApp
is wrapped with ProviderScope
, making the providers available.MyHomePage
uses ConsumerWidget to easily access providers.ref.watch(counterProvider)
listens to changes in the counterProvider
and rebuilds the widget when the counter value updates.ref.read(counterProvider.notifier).state++
reads the provider and increments the state, triggering a rebuild due to ref.watch.Benefits of Riverpod:
- Improved Performance: Riverpod's precise rebuilds and avoidance of unnecessary widget updates contribute to better app performance.
- Enhanced Code Structure: Riverpod promotes a cleaner separation of concerns, making your code more organized and maintainable.
- Simplified Testing: Mocking and testing state logic becomes significantly easier with Riverpod's test-friendly architecture.
Riverpod offers a robust and scalable solution for state management in Flutter. Its compile-time safety, improved testability, and streamlined access to state make it an excellent choice for projects of all sizes. By understanding the core concepts of providers, ref
, and scoping, you can leverage the power of Riverpod to build more maintainable, performant, and testable Flutter applications.
Flutter BLoC
Flutter BLoC is a powerful state management library for Flutter applications. It is simple to use and understand, and it has good documentation and many examples. Flutter BLoC is also powerful and can be used to create all kinds of applications, from simple to complex. It is also easy to test your bloc logic.
What is BLoC?
BLoC stands for Business Logic Component. It is a state management library that helps you to separate your application's business logic from its user interface. This makes your code more modular, testable, and maintainable.
How does BLoC work?
BLoC works by using three main components:
- Events: Events are used to communicate with the BLoC from the user interface. For example, a button click could be an event.
- States: States are used to store the current state of the application. For example, the state of a counter could be the number of times it has been clicked.
- BLoCs: BLoCs are responsible for processing events and emitting new states.
How to use BLoC
To use BLoC in your Flutter application, you need to create a new BLoC class. The BLoC class should extend the Bloc
class and implement the mapEventToState
method. The mapEventToState
method is used to process events and emit new states.
Once you have created your BLoC class, you can use the BlocProvider
widget to make your BLoC available to the rest of your application. The BlocProvider
widget takes two arguments: a BLoC class and a function that creates a new instance of the BLoC class.
You can then use the BlocBuilder
widget to listen to state changes from your BLoC. The BlocBuilder
widget takes two arguments: a BLoC class and a function that builds the UI based on the current state.
Example
Here is a simple example of how to use BLoC in a Flutter application:
import 'package:flutter/material.dart';
import 'package:flutter_bloc/flutter_bloc.dart';
class CounterEvent {}
class CounterState {
final int count;
CounterState(this.count);
}
class CounterBloc extends Bloc {
CounterBloc() : super(CounterState(0));
@override
Stream mapEventToState(CounterEvent event) async* {
final currentState = state;
if (event is CounterEvent) {
yield CounterState(currentState.count + 1);
}
return currentState;
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: BlocProvider(
create: (context) => CounterBloc(),
child: MyHomePage(),
),
);
}
}
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: BlocBuilder(
builder: (context, state) {
return Text('Counter: ${state.count}');
},
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
context.read().add(CounterEvent());
},
child: Icon(Icons.add),
),
);
}
}
In this example, we have created a new BLoC class called CounterBloc
. The CounterBloc
class has two states: CounterState
and CounterEvent
. The CounterState
class has a single property called count. The CounterEvent
class is empty.
The mapEventToState
method of the CounterBloc
class is used to process events and emit new states. In this case, the only event that we are handling is the CounterEvent
event. When we receive a CounterEvent
event, we increment the count
property of the current state and emit a new CounterState
object.
The MyApp
widget uses the BlocProvider
widget to make the CounterBloc
available to the rest of the application. The MyHomePage
widget uses the BlocBuilder
widget to listen to state changes from the CounterBloc
. The BlocBuilder
widget builds the UI based on the current state.
Benefits of BLoC
There are many benefits to using BLoC in your Flutter applications. Some of the benefits include:
- Modularity: BLoC helps you to separate your application's business logic from its user interface. This makes your code more modular and easier to test.
- Testability: BLoC makes it easy to test your business logic without having to worry about the user interface.
- Maintainability: BLoC makes your code more maintainable by making it easier to understand and modify.
BLoC is a powerful and flexible state management library for Flutter. It is simple to use and understand, and it has good documentation and many examples. BLoC is also powerful and can be used to create all kinds of applications, from simple to complex. It is also easy to test your bloc logic.
What is best for me?
Choosing the right state management solution for your Flutter application depends on your specific needs and requirements. If you are building a small to medium-sized application with basic state management needs, Provider is a good choice. If you are building a large application with complex state management needs, Riverpod or BLoC may be a better fit.
Here is a table summarizing the key differences between the three solutions:
Feature | Provider | Riverpod | BLoC |
---|---|---|---|
Learning curve | Easy | Moderate | Difficult |
Performance | Good | Excellent | Good |
Testability | Good | Excellent | Excellent |
Boilerplate code | Minimal | Moderate | Extensive |
Use cases | Small to medium-sized apps | Large apps with complex state | Large apps with complex business logic |
Provider
Provider is a simple and easy-to-learn state management solution that is officially recommended by the Flutter team. It is based on the concept of dependency injection, where dependencies are provided to widgets through a hierarchical tree structure. Provider makes it easy to access and update data anywhere in your widget tree, without the need for complex boilerplate code.
ProvidStrengths of Provider:
- Easy to learn and use: Provider has a simple API and requires minimal boilerplate code, making it easy for beginners to get started with state management in Flutter.
- Good for small to medium-sized apps: Provider is a good choice for applications that don't require complex state management logic.
- Officially recommended by Flutter: Provider is the recommended state management solution by the Flutter team, which means it is well-supported and maintained.
Weaknesses of Provider:
- Can become complex for large apps: As your application grows, Provider can become difficult to manage, especially if you have a lot of dependencies.
- Not as performant as other solutions: Provider can have performance issues in large applications with complex state updates.
Use cases for Provider:
- Simple applications with basic state management needs
- Small to medium-sized apps
- Projects where ease of use and quick development are priorities
Riverpod
Riverpod is a state management solution that is built on top of Provider. It aims to address some of the limitations of Provider by providing compile-time safety, improved performance, and better testability. Riverpod introduces the concept of "providers", which are objects that hold and manage the state of your application.
Strengths of Riverpod:
- Compile-time safety: Riverpod provides compile-time safety, which means that errors related to state management can be caught during development, rather than at runtime.
- Improved performance: Riverpod is designed to be more performant than Provider, especially in large applications with complex state updates.
- Better testability: Riverpod makes it easier to write unit tests for your state management logic.
Weaknesses of Riverpod:
- Steeper learning curve: Riverpod has a more complex API than Provider, which can make it more difficult to learn.
- Requires more boilerplate code: Riverpod requires more boilerplate code than Provider, which can make it less appealing for small projects.
Use cases for Riverpod:
- Large applications with complex state management needs
- Projects where performance and testability are critical
- Applications that require compile-time safety
BLoC (Business Logic Component)
BLoC is a state management pattern that is based on the concept of separating business logic from the user interface. BLoC uses events and states to manage the flow of data in your application. BLoC is a good choice for large applications with complex business logic.
Strengths of BLoC:
- Clear separation of concerns: BLoC enforces a clear separation between business logic and the user interface, which makes your code more maintainable and testable.
- Good for complex business logic: BLoC is a good choice for applications with complex business logic that requires a lot of state manipulation.
Weaknesses of BLoC:
- Steeper learning curve: BLoC has a more complex architecture than Provider or Riverpod, which can make it more difficult to learn.
- Requires more boilerplate code: BLoC requires more boilerplate code than Provider or Riverpod, which can make it less appealing for small projects.
Use cases for BLoC:
- Large applications with complex business logic
- Projects where maintainability and testability are critical
- Applications that require a clear separation of concerns
Conclusion
Choosing the right state management solution for your Flutter application depends on your specific needs and requirements. If you are building a small to medium-sized application with basic state management needs, Provider is a good choice. If you are building a large application with complex state management needs, Riverpod or BLoC may be a better fit.
References:
Provider:
- Provider Package on pub.dev - Official Documentation
- Flutter Provider: What Is It, What Is It For, and How to Use It? - Medium
- Flutter Provider Package - GeeksforGeeks